Table of Contents
Introduction to Where function in Numpy
Numpy returns the element based on the condition (i.e Logical Condition) Which we are passing into the np.where function.
Syntax of where function in NumPy is:-
np.where()
Attribute in where function:-
np.where( condition , return if true, return if false )
condition:- here we pass logical condition
return if true:- if the condition is true then return the value of first place
Return if false:- if the condition is false then return the value of the second place
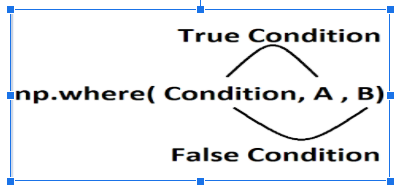
Whereas,
If the condition is true then return value of A array
If the condition is false then return the value of B array
Code:-
import numpy as np
A = np.array([10,20,30,40,50])
B = np.array([100,200,300,400,500])
condition = np.array([True,True,False,False,False])
print("First Array :: ",Array_one)
print("Second Array :: ",Array_two)
print("Logical conditional array :: ",condition)
output_where = np.where(condition, A, B) # Here we apply where function
print("\n Output of Where Function :: ",output_where)
Output:-
First Array :: [10 20 30 40 50]
Second Array :: [100 200 300 400 500]
Logical conditional array:: [ True True False False False]
Output of Where Function :: [ 10 20 300 400 500]
Example 2:- pass the condition is the values is greater than 2 then return true otherwise false
import numpy as np
A = np.array([10, 20, 30, 40, 50])
np.where( A > 20, 'YES', 'NO')
OUTPUT:-
array(['NO', 'NO', 'YES', 'YES', 'YES'], dtype='<U3')
Example 4:- Apply were function on the 2D array.
AA = np.arange(1,5).reshape(2,2)
print('First Array : \n',AA)
BB = np.arange(20,51,10).reshape(2,2)
print('Second Array \n',BB)
CC = np.array([[True, False],[False,True]])
print('Conditional Array : \n',CC)
Output = np.where(CC,AA,BB)
print('\n Output Of Where Function : \n',Output)
# Here we pass the first condition is store in CC
# Then AA array(i.e If the condition is True then return AA value)
# Then BB array(i.e If the condition is False then return BB value)
Output:-
First Array :
[[1 2]
[3 4]]
Second Array
[[20 30]
[40 50]]
Conditional Array :
[[ True False]
[False True]]
Output Of Where Function :
[[ 1 30]
[40 4]]
Example 5:-
AA = np.arange(10,50,10).reshape(2,2)
print('First Array : \n',AA)
BB = np.arange(100,500,100).reshape(2,2)
print('Second Array \n',BB)
CC = np.array([[True, False],[False,True]])
print('Conditional Array : \n',CC)
Output = np.where(CC,AA,BB)
print('\nOutput Of Where Function : \n',Output)
# Here we pass the first condition is store in CC
# Then AA array(i.e If the condition is True then return AA value)
# Then BB array(i.e If the condition is False then return BB value)
Output:-
First Array :
[[10 20]
[30 40]]
Second Array
[[100 200]
[300 400]]
Conditional Array :
[[ True False]
[False True]]
Output Of Where Function :
[[ 10 200]
[300 40]]
Finding the indices value in the numpy array
Using np.where function we get the index value of an array.
Example:-
import numpy as np
# Create a numpy array from a list of numbers
arr = np.array([10, 12, 15 ,10, 14 ,11, 12, 15, 18 , 10])
# Get the index of elements with value 10
result = np.where(arr == 10)
print('Array value : ',arr)
print('Elements with value 10 exists at following indices : ', result)
Example 2:- Access the 2D Array indices value
# Create a 2D Numpy array from list of lists
arr = np.array([[11, 12, 13],
[14, 10, 16],
[17, 10, 11],
[12, 14, 10]])
print('2D Array value is : \n ',arr)
# Get the index of elements with value 10
result_2D = np.where(arr == 10)
print('Elements with value 10 exists at following indices : \n ', result_2D)
Output:-
2D Array value is :
[[11 12 13]
[14 10 16]
[17 10 11]
[12 14 10]]
Elements with value 10 exists at following indices :
(array([1, 2, 3], dtype=int64), array([1, 1, 2], dtype=int64))
Finding the indices value using a logical operator
In this part, we apply the logical operator which is AND and OR
Rule of AND( & )
True & True = True
False & True = False
True & False = False
False & False = False
Rule of OR( & )
True | True = True
False | True = True
True | False = True
False | False = False
Example:- Apply AND Operator
import numpy as np
# Create a numpy array from a list of numbers
arr = np.array([10, 12, 15 ,10, 14 ,11, 12, 15, 18 , 10])
# Get the index of elements with value 10
result = np.where((arr > 10) & (arr < 15))
print('Array value : ',arr)
print('Elements with value in between 10 and 15 exists at following indices : \n', result)
Output:-
Array value : [10 12 15 10 14 11 12 15 18 10]
Elements with value in between 10 and 15 exists at following indices :
(array([1, 4, 5, 6], dtype=int64),)
Example:- Apply Or Operator
import numpy as np
# Create a numpy array from a list of numbers
arr = np.array([9, 10, 12, 15 ,10, 5,11, 12, 15, 18 , 10])
# Get the index of elements with value 10
result = np.where((arr < 10) | (arr > 15))
print('Array value : ',arr)
print('Elements with value in less than 10 and greater 15 exists at following indices: \n', result)
Output:-
Array value : [ 9 10 12 15 10 5 11 12 15 18 10]
Elements with value in less than 10 and greater 15 exists at following indices :
(array([0, 5, 9], dtype=int64),)
Conclusion
In this blog get you the understanding of how to use numpy where function and how to access the indices using where function and its syntex.Operations on 1D array And 2D array and the working process of where function.